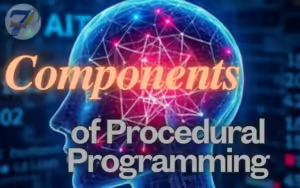
Procedural programming is the combination of different components. Those components help design and emphasize creating code that is understandable, modular, and manageable through different processes. This is an essential approach for the maintainability and scalability of effective projects. In this article, we will get an overview of different components of procedural programming. components of this design process include data types, variables, data structures, control flow, functions, and subroutines.
Components of Procedural Programming
1. Variable
Variables are the essential component of procedural programming. It is the symbolic representation that stores information that can change throughout a program’s execution. The data stored in variables is defined by data types. Here’s a brief overview of variable types:
-
Integer
Integer type holds whole numbers; in other words, it stores numeric values without decimal points, e.g., int number {10}.
-
Float
Floating point represents decimal values e.g. float numbers{10.5};
-
Char
Character data type is used to store single character e.g. char grade{“A”};
-
Character Array
Used for strings e.g. char string[10]{“nadirahmad”}
Initializing a variable refers to assignment of an initial value at the time of decleration, that can be modify later.
2. Data Structures
Data structures refer to another important component that allows the organization and management of data in procedural programming as well as other languages. They allow for complex data manipulation and operations.
- Arrays: Collections of elements of the same type of store in contiguous memory, e.g., int 10] {1, 2, 3, 4,5};
Additionally, the size of the array must be defined; it should be a constant integral number, while in the case of a dynamic array, the size can be input by the user during runtime, and in a c-stings (character array), the size is determined after initialization.
- Structures: Allow to group out various data types together in procedural programming (e.g. a structure to represent a student).
Choosing the right data structure is crucial for performance. Arrays work well for large datasets, while structures are beneficial for more complex data relationships.
3. Control Flow
Control flow is just like a doctor that allows and denies the patient from different situations. It determines the order in which the instructions execute; in other words, it controls the order of execution, enabling programs to show dynamic responses in different situations.
- Conditional Statements: This component of procedural programming executes specific blocks of code based on conditions. It has different categories, including if, if else, and switch statements.
simple usage of conditional statement written in C++ language is given belowint age{25};if (age<18)cout<<“teenage”;elsecout<<“adultt”;
-
Loops:
Loops are another important component in procedural programming that are used to repeat different blocks of code according to the situation. There are three different types of loops, including for loop, while loop, and do while loop.
- For Loop:
Executes a block a specific number of times. For loop use when the actual number of executions is known, it means when we confirmed how many times the sort of code will run.
for(int index{1};index<=5;index++)
cout<<“hello world”;
In this example, we know that the number of executions of code is 5, so we use for loop.
- While Loop:
Continues until the condition is false. It is used when the number of iterations is not confirmed.
char ch{a}; while(ch!=’z’) cout<<ch; ch++;
- Do-While Loop:
- Executes at least once before checking the condition. It is also called a posttest loop, in which it is tested after the body of the loop.
4. Functions
Functions are another important components of procedural programming that refer to blocks of code designed to perform specific tasks .Functions are reuseable that enhance the modularity and readability of the program.
C++
int sum(int num1,int num2)
return num1+num2;
int main ()
{
int sum=sum(2,2);
}
Benefits of Functions:
- Modularization: It allow to breakdown the code to manage effectively.
- Reusability: Functions can be called from different parts of the program.
- Manageability: Changes can be made in one location without affecting the entire program.
5. Subprograms
Subprograms are another main component of programming that include both functions and procedures, designed to perform specific tasks. While functions return a value, procedures typically do not.
Benefits of Subprograms:
- Encourage organization and reduce code repetition.
- Allow developers to focus on higher-level processes.
Conclusion
A solid understanding of these components—variables for dynamic data management, data structures for organizing information, control flow for decision-making, and functions/subprograms for modularity—forms the foundation for effective programming.
read more about procedural programming click here
read more about data types
FAQs
What are variables in procedural programming?
Variables are symbolic representations that store data, which can change during program execution. Common types include integers, floats, characters, and character arrays.
What role do data structures play?
Data structures organize and manage data effectively. Common types include arrays (for collections of the same type) and structures (for grouping different data types).
How does control flow work?
Control flow determines the execution order of instructions using conditional statements (like if-else) and loops (for, while, and while) to control program behavior based on conditions.
What are functions, and why are they important?
Functions are reusable blocks of code that perform specific tasks, enhancing modularity, readability, and manageability of programs by allowing code reuse.
What are subprograms?
Subprograms include both functions and procedures. Functions return values, while procedures do not, both helping to reduce code repetition and improve organization in programming
Progress requires perseverance and a constant effort to make the blog error-free for improved user experience and bots. This blog needs to be updated because h2 (variables) has at least 20 typical grammar mistakes.
your concideration means a world for our progress.