Did you know Google declared Kotlin the official language for developing Android apps? Therefore, mastering the basics of this programming language is necessary for efficient Android app development. It enhances productivity because it has concise syntax and works smoothly with Java.
// comparison
// Java:
public class HelloWorld { public static void main(String[] args)
{ System.out.println("Hello World"); }
}
// Kotlin:
fun main() = println("Hello World")
But it’s not just about coding; it’s about unlocking secrets that can elevate your projects from ordinary to extraordinary. Understanding the basics of the Kotlin programming language can increase your workflow and improve app performance.
What is Kotlin?
It is an open-source, statically typed programming language developed by JetBrains. It has both the features of object-oriented programming (OOP) and functional programming.
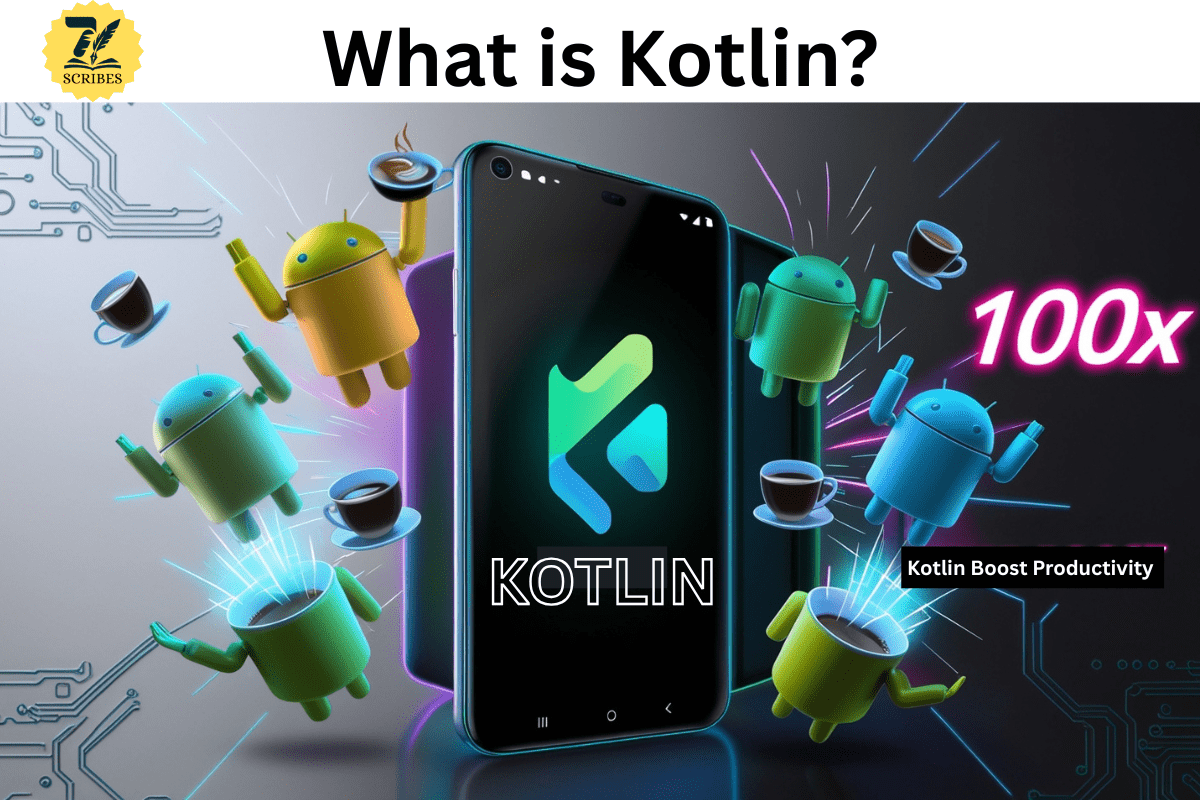
// OOP example class
Car(val model: String)
{ fun drive() = println("Driving $model")
}
// Functional example
val numbers = listOf(1, 2, 3)
val doubled = numbers.map { it * 2 }
History:
The development of the Kotlin language was announced in the year 2011. It was released in February 2016.
In 2017, Google announced first-class support for Kotlin on Android. Later, Kotlin 1.2 was released with features like code sharing between JVM and JavaScript.
After that, different versions of Kotlin were released, such as 1.3 in 2018, 1.4 in 2020, 1.5 in 2021 etc.
Why it is Great for Android Development
It is one of the fastest-growing and widely used programming languages. In May 2017, Google announced it as an official language for developing Android applications.
It has become a popular choice for building Android apps. It helps code quickly and neater than other languages. Its benefits include:
1. Clean and Short Code
It enables you to write less code to do more things. It suggests:
// Data class example (auto-generates toString(), equals(), etc.)
data class User(val name: String, val age: Int)
// vs Java equivalent which would require 50+ lines
- Fewer errors in your code
- Easier to read and update later
- Avoid repetition of the self-evident (e.g., variable types)
2. No More Null Errors
In Java, a “null pointer” exception crashes apps frequently. It fixes this by:
- In this programming language, variables are non-nullable by default.
- Using “?” when null is necessary
- Preventing crashes before they occur
val nonNull: String = "Hello" // Compiler enforces
non-nullval nullable: String? = null // Must be explicitly nullable
// Safe calls
val length = nullable?.length ?: 0
3. Works with Java Easily
You don’t have to rewrite old Java code to use it because:
- It works together with Java smoothly
- Add it to existing projects bit by bit
- Keep using Java libraries while trying Kotlin
// Calling java
val javaList = ArrayList<String>() // Java ArrayList
javaList.add("it works!")
// Calling it from Java
@file:JvmName("Utils")
fun greet() = "Hello "
// Java file
:String message = Utils.greet();
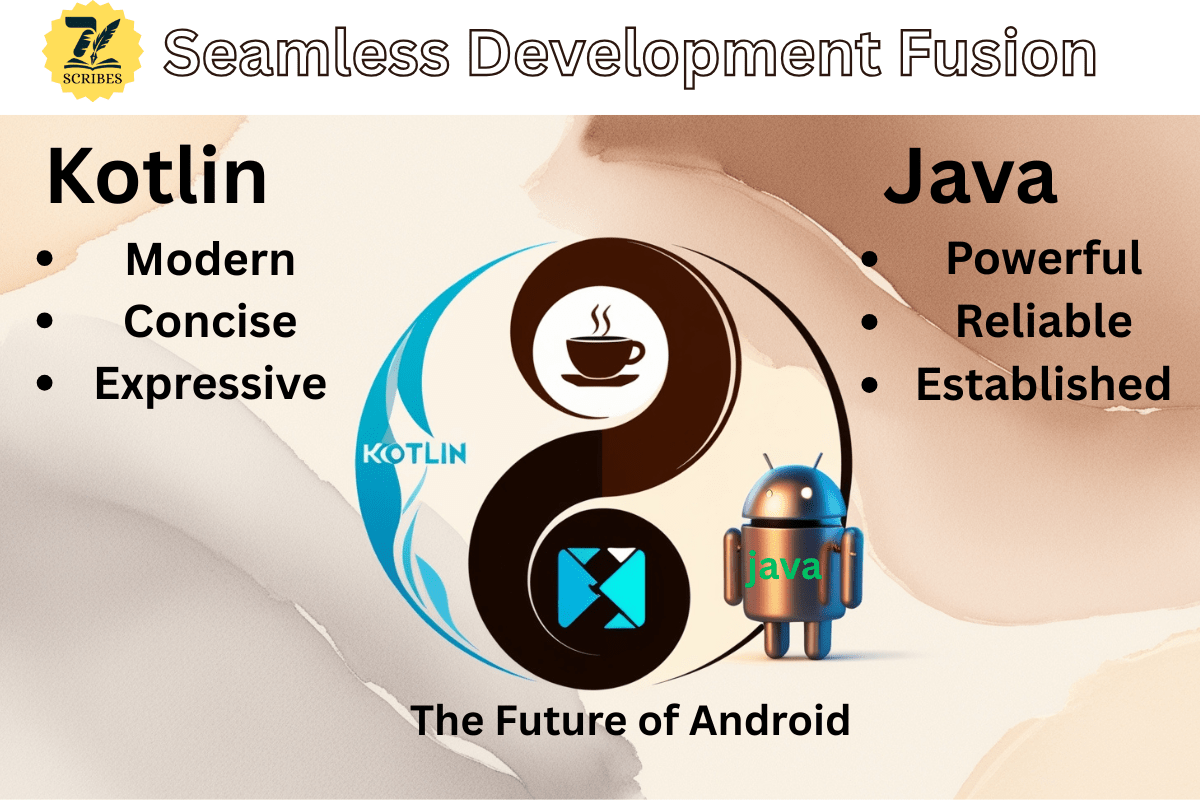
Setting Up Your Kotlin Development Environment
Before coding in Kotlin, it is essential to install a friendly development environment (IDE). To create an effective workflow, do the following:
1. Choose a proper IDE
You can begin your Kotlin coding experience from any of the following IDE
- Android Studio (Version 4.0+): The official IDE for Android development with built-in Kotlin support
- IntelliJ IDEA (Community or Ultimate Edition): JetBrains’ top-of-the-line IDE with great Kotlin support
- Visual Studio Code (With Kotlin extensions): A lightweight alternative supporting plugins
2. Installation and Configuration
Install your chosen IDE based on the official installation manual.
For VS Code or earlier IntelliJ versions, install the Kotlin plugin:
Open Marketplace/Plugins → Search for “Kotlin” → Install
Set JDK (Java Development Kit) 8 or later
3. Check Your Setup
Make a test project and execute this minimal program:
fun main() { println("Start your journey today!")}
4. Recommended Additional Tools
- Kotlin Playground: For rapid experiments (play.kotlinlang.org)
- Kotlin CLI Compiler: For compilation from the command line
Best Practices for Optimal Setup
- Keep regular IDE updates for new Kotlin features
- Utilize built-in tools like code completion and refactoring support
- Consider version control integration from the outset
For comprehensive setup instructions, consult the official Kotlin documentation: kotlinlang.org/docs
Core Concepts for Modern Android Coding
Understanding Kotlin’s syntax and core concepts is crucial for writing effective Android applications. Kotlin’s syntax is expressive and concise, which reduces boilerplate code and enhances readability.
For example, we can use the val keyword to define read-only variables. We use var for variables that can change. This not only makes the code more readable but also enforces immutable where necessary. This leads to safer and more predictable code.
val immutableList = listOf(1, 2, 3) // Read-only
var mutableCounter = 0 // Mutable
In this module, we will see some core concepts of this programming language.
1. Clean Code Structure
- Immutable references use simple declarations
- Mutable variables have straightforward syntax
- Achieve more functionality with less typing compared to traditional options
2. Powerful Built-in Features
- Smart class types automatically generate standard methods
// Automatic equals(), hashCode(), toString(), etc.
data class Person(val name: String, val age: Int)
- Compact function expressions enable cleaner callback patterns
button.setOnClickListener { view -> println("Button clicked!")}
3. Program Flow Control
- Conditional blocks can directly return results
Val max = if (a > b) a else b
- The advanced case statement handles complex scenarios elegantly
when (x) { 1 -> print("One") in 2..10 -> print("Between 2 and 10") else -> print("Other")}
- Loop structures maintain familiar patterns with improved readability
for (i in 1..10) { println(i) }
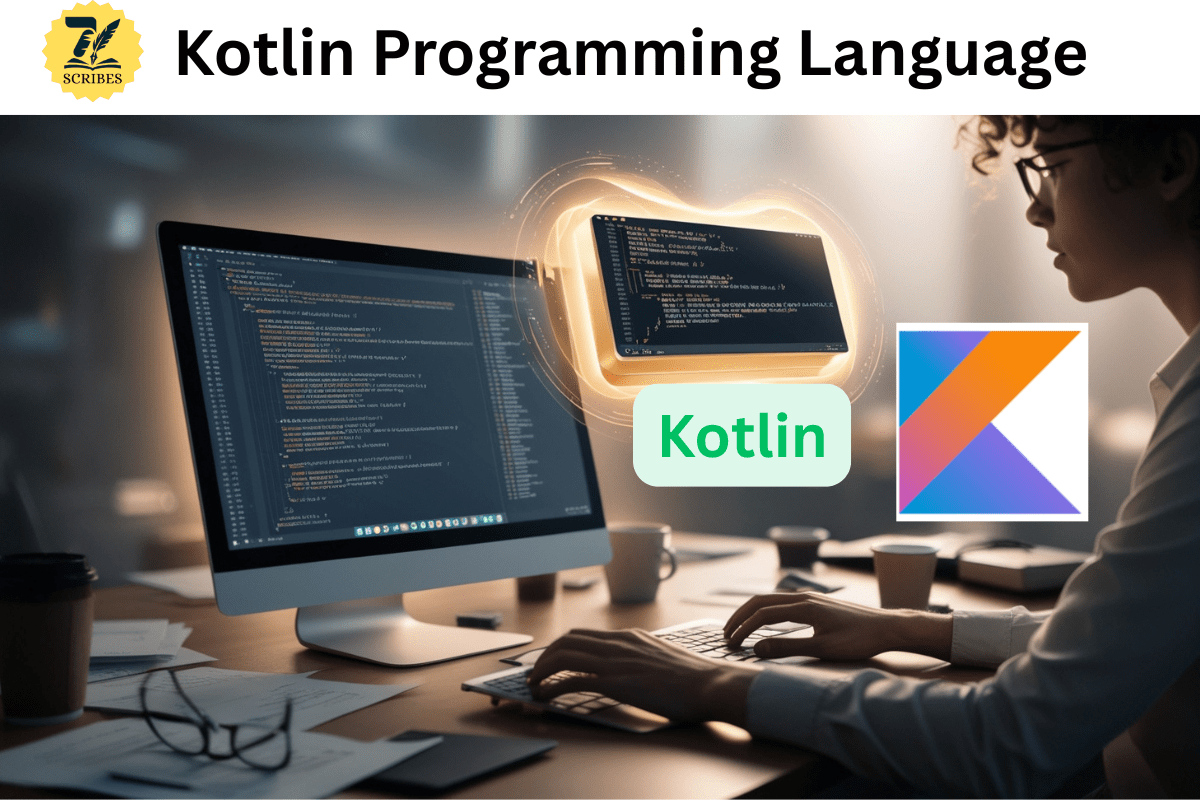
Powerful Features to Write Better Code
Once you complete and become proficient in the core concept. Then start learning advanced Kotlin concepts. This will improve your efficiency. Some advanced concepts are:
1. Coroutines & Structured Concurrency
viewModelScope.launch { val data = withContext(Dispatchers.IO) { fetchData() }
updateUI(data)
}
2. Kotlin Flow (Cold Streams)
fun getUsers(): Flow<List<User>> = flow
{
emit(api.fetchUsers())
}
3. Delegated Properties (by keyword)
val lazyValue: String by lazy {
println("Computed!")
"Hello"}
4. Sealed Classes & Interfaces
sealed class Result<out T> {
data class Success<T>(val data: T) : Result<T>()
data class Error(val message: String) : Result<Nothing>()
}
5. Inline & Reified Generics
inline fun <reified T> parseJson(json: String): T
{
return Gson().fromJson(json, T::class.java)
}
6. Type-Safe Builders (DSLs)
html { head { title(" DSL") }}
7. Context Receivers (Kotlin 1.7+)
context(View, CoroutineScope)fun updateUI() { ... }
8. Multiplatform (KMM)
// Shared module
expect fun platformMessage(): String
// Android implementation
actual fun platformMessage() = "Hello Android!"
9. Deep Recursion with TailRec
tailrec fun factorial(n: Int, acc: Int = 1): Int
{
return if (n == 1) acc else factorial(n - 1, acc * n)
}
10. Contracts (Compiler Hints)
fun String?.isValid(): Boolean {
contract {
returns(true) implies (this@isValid != null)
}
return !isNullOrEmpty()
}
1. Adding New Features to Existing Classes
You can give extra abilities to any class without changing its original code.
// Add extension to String class
fun String.isValidEmail(): Boolean
{
return contains("@") && endsWith(".com")
}
- Works even with classes from other libraries
- Example: Add an isValidEmail() check to Strings
- Makes your code neater and easier to reuse
2. Handling Background Tasks Smoothly
Manage multiple operations at once without complicated callbacks.
suspend fun fetchUserData(): User {
return withContext(Dispatchers.IO) {
api.getUser() // Runs in background }
}
- Write code that looks simple but works in the background
- Use suspend for functions that can pause and continue
- Keeps your app running fast while doing heavy work
3. Smarter Ways to Manage Data
Control how your variables behave when used or changed.
var observedValue by Delegates.observable(0) { _, old, new ->
println("Changed from $old to $new")}
- Lazy loading: Only sets up when first needed (saves time)
- Change tracking: Runs code automatically when values are updated
- Safe changes: Check if updates are valid before applying
Why These Tools Help
- Do more with less code
- Fewer mistakes in complex tasks
- Apps run faster and smoother
- Easier to update and improve later
Adding Kotlin to Your Current Java Projects
If you are working on a project in which you are writing code in Java and you want to shift towards Kotlin, you don’t need to write all the code you have already written. You can write Kotlin in Java projects because they are compatible with each other.
1. Mixing Both Languages Smoothly
You don’t need to rewrite everything at once. Start small:
// Java class
public class JavaClass { public static String greet() {
return "Hello";
}
}
{
println(JavaClass.greet()) // Works seamlessly
}
- Write new features in Kotlin
- Keep existing Java code as-is
- Both languages work together without problems
2. Helpful Tools for Compatibility
Special annotations make the transition easier:
- @JvmName: Changes how Kotlin functions appear in Java
- @JvmOverloads: Helps Java use Kotlin’s default values
- These keep your code working smoothly across both languages
fun showGreeting() {
println(JavaClass.greet()) // Works seamlessly
}
3. Converting Java Code Step by Step
Android Studio can help switch existing files:
- Right-click Java files → “Convert to Kotlin”
- Clean up the result to match Kotlin’s best practices
- Focus on one part at a time to avoid breaking things
Why This Approach Works
- No need to stop current work for a full rewrite
- Get Kotlin’s benefits where it matters most
- Your team can learn gradually
- The whole project becomes cleaner over time
Best Practices
In this module, you will learn the best practices while writing the code. So that you become the top 1% coder. Here are some best practices you should follow:
1. Keep Your Code Short and Clear
// Prefer val over var
val PI = 3.14159
//Immutable
var counter = 0
// Mutable only when necessary
// Use data classes for models
data class User(val id: Int, val name: String)
- Use a data class for simple objects (auto-generates useful methods)
- Prefer val over var when values don’t change (fewer bugs)
- Let the language’s smart features do the work for you
2. Extend Without Changing
Add new features to existing classes with extension functions:
// RecyclerView extension
fun RecyclerView.setup(adapter: Adapter<*>)
{ this.adapter = adapter
layoutManager = LinearLayoutManager(context)
}
- Add new features to existing classes with extension functions
- Example: Create a setupRecyclerView() extension to avoid repeating code
- Use lambdas to write cleaner callbacks and operations
3. Handle Null Values Safely
val nullableString: String? = getPossibleNull()
// Safe calls
nullableString?.let {
println(it.length) }
// Elvis operator
val length = nullableString?.length ?: 0
- Avoid nulls when possible (use regular types instead)
- For cases where nulls are needed:
- Use ?.let { } for safe operations
- Try to apply/also for working with nullable objects
- run/ helps group-related operations cleanly
Why These Matter
- Easier to read: Others (and future you) will understand quickly
- Fewer crashes: Proper null handling prevents common errors
- Less typing: Do more with less code
- Simpler updates: Well-organized code is easier to change later
Essential Kotlin Tools for Android Development
In this module, we will explore some essential tools that you can try.
1. Koin: Simplified Dependency Injection
Lightweight alternative to Dagger.
val appModule = module {
single { NetworkService() }
factory { Presenter(get()) }
}
- Uses Kotlin DSL instead of complex annotations
- Easy setup with clear dependency declarations
- Improves code organization and testability
- Best for: Projects needing clean architecture without DI complexity
2. Anko: JetBrains’ Android Toolkit
(Note: Consider Jetpack Compose for new projects)
verticalLayout {
button("Click me") {
onClick { toast("Hello!") }
}
}
- Replace XML layouts with Kotlin DSL
- Built-in helpers for activities, dialogs, and SQLite
- Simplifies common tasks with intuitive syntax
3. Coroutines & Flow: Modern Async Handling
viewModelScope.launch
{
val user = async { getUser() }
val posts = async { getPosts() }
updateUI(user.await(), posts.await())
}
- Coroutines replace callbacks with sequential-style code
- Flow enables reactive data streams
- Built into Kotlin (no additional dependencies)
- Handles network calls, DB operations, and more
- Key Benefit: Prevents callback hell while maintaining performance
Why These Matter?
- Faster development with less boilerplate
- More reliable apps through structured concurrency
- Easier maintenance with organized code
- Seamless integration with existing Java code
These tools represent Kotlin’s philosophy of pragmatic, developer-friendly solutions. Each solves specific pain points in Android development while maintaining interoperability with existing Java codebases.
Kotlin in the Real World: Success Stories
1. Pinterest’s Transformation
- Challenge: Needed cleaner code and fewer crashes
- Solution: Switched from Java to Kotlin
- Results:
- 30% reduction in null pointer exceptions
- More readable code attracted top talent
- Faster feature development
“Kotlin’s null safety alone saved us hundreds of crash reports weekly” – Pinterest Android Team
2. Trello’s Gradual Adoption
- Approach: Mixed it with existing Java code
- Key Benefits:
- Coroutines simplified background tasks
- Extension functions reduced duplicate code
- Smooth transition with zero downtime
3. Evernote’s Modernization
- Improvements:
- 40% less boilerplate code
- Functional programming made logic clearer
- Significant drop in production crashes
Why These Companies Chose it?
- For Teams: Happier developers, faster onboarding
- For Business: More stable apps, quicker updates
- For Users: Smoother experience, fewer crashes
The Future of Kotlin
What’s Next?
- Kotlin Multiplatform
- Share code across Android, iOS, and web
- Write once, deploy everywhere
- Already used by Netflix, VMware
// Shared module
expect fun platformMessage(): String
// Android implementation
actual fun platformMessage() = "Hello Android"
- Growing Job Market
- 65% more jobs for this programming language since 2022
- Now required for most Android roles
- New Features Coming
- Even better performance
- More tools for large teams
- Enhanced debugging
Getting Started with Kotlin
First Steps:
- Try it in one module of your app
- Learn coroutines for background tasks
- Explore multiplatform for future projects
Resources:
- Official Kotlin docs (free)
- Google’s Kotlin courses
- Active community forums
“The best time to learn it was yesterday. The second best time is now.” – Senior Android Developer, Spotify.
Final Thought
It isn’t just another language, it’s becoming the standard for building better Android apps faster. From startups to tech giants, teams are seeing real benefits in quality, speed, and developer satisfaction. The question isn’t whether to adopt it, but how soon you can start.
fun main() {
println("Start your journey today!")
}
Q1) What is Kotlin used for?
It is a modern programming language mainly used for Android app development. It is also used for backend development and cross-platform apps.
Q2) Is kotlin like Python?
No, Kotlin is not as concise as Python, but it’s much shorter than Java while being more structured and type-safe.
Q3) Is Kotlin difficult to learn?
No, kotlin is not difficult to learn, especially if you already know about Jave. Because both languages are compatible with each other.
Read More about: